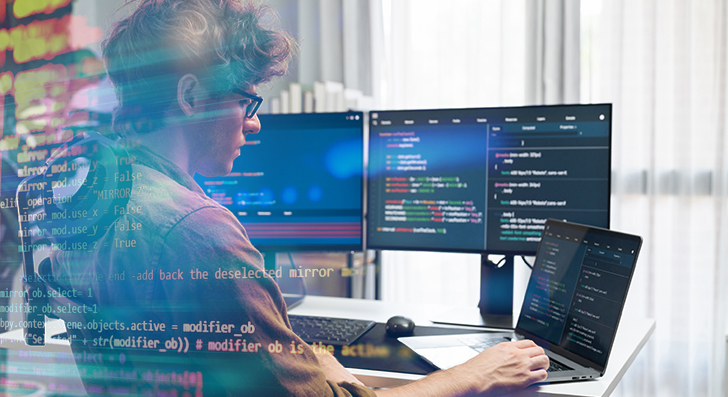
Scalability usually means your application can manage growth—more people, far more information, and more traffic—devoid of breaking. Being a developer, developing with scalability in your mind saves time and worry later on. Here’s a transparent and sensible guideline that may help you commence by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability isn't a thing you bolt on later—it ought to be component of your respective strategy from the beginning. A lot of applications fall short when they increase fast due to the fact the original layout can’t handle the extra load. For a developer, you have to Assume early about how your technique will behave stressed.
Begin by planning your architecture to generally be flexible. Prevent monolithic codebases where almost everything is tightly related. Instead, use modular layout or microservices. These styles crack your app into smaller sized, impartial pieces. Every module or provider can scale By itself without affecting The entire process.
Also, give thought to your databases from working day 1. Will it need to have to manage one million users or simply a hundred? Select the appropriate kind—relational or NoSQL—determined by how your facts will mature. Plan for sharding, indexing, and backups early, even if you don’t require them but.
One more critical place is to prevent hardcoding assumptions. Don’t compose code that only operates beneath recent problems. Contemplate what would transpire If the person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use layout designs that help scaling, like message queues or occasion-driven systems. These help your app cope with additional requests devoid of having overloaded.
When you build with scalability in mind, you are not just planning for achievement—you might be lessening upcoming problems. A properly-planned method is easier to maintain, adapt, and mature. It’s superior to prepare early than to rebuild afterwards.
Use the appropriate Database
Selecting the right databases is often a critical Section of creating scalable applications. Not all databases are developed exactly the same, and utilizing the Mistaken one can gradual you down and even cause failures as your application grows.
Commence by understanding your info. Is it remarkably structured, like rows in a very table? If Sure, a relational databases like PostgreSQL or MySQL is an efficient fit. These are definitely sturdy with relationships, transactions, and regularity. Additionally they support scaling approaches like study replicas, indexing, and partitioning to take care of a lot more traffic and knowledge.
If your knowledge is a lot more adaptable—like user action logs, product catalogs, or paperwork—take into consideration a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at handling massive volumes of unstructured or semi-structured data and may scale horizontally much more simply.
Also, consider your go through and produce patterns. Do you think you're accomplishing plenty of reads with less writes? Use caching and skim replicas. Are you dealing with a hefty publish load? Take a look at databases that may take care of significant write throughput, or perhaps function-dependent details storage methods like Apache Kafka (for non permanent data streams).
It’s also intelligent to Feel forward. You might not will need Highly developed scaling features now, but choosing a database that supports them implies you gained’t have to have to modify afterwards.
Use indexing to hurry up queries. Avoid pointless joins. Normalize or denormalize your information according to your accessibility designs. And often check database general performance when you mature.
To put it briefly, the ideal databases will depend on your application’s framework, pace wants, And the way you assume it to mature. Choose time to select correctly—it’ll preserve plenty of difficulty later.
Improve Code and Queries
Rapid code is vital to scalability. As your app grows, each modest delay adds up. Improperly published code or unoptimized queries can slow down overall performance and overload your system. That’s why it’s important to Establish successful logic from the start.
Begin by crafting clean, very simple code. Prevent repeating logic and remove anything avoidable. Don’t select the most complicated Alternative if an easy 1 works. Maintain your functions brief, concentrated, and simple to check. Use profiling equipment to locate bottlenecks—sites the place your code takes far too extended to operate or works by using an excessive amount memory.
Subsequent, evaluate your database queries. These normally sluggish matters down a lot more than the code alone. Ensure each query only asks for the info you actually will need. Prevent Choose *, which fetches anything, and rather pick out specific fields. Use indexes to speed up lookups. And stay clear of performing a lot of joins, especially across substantial tables.
If you see exactly the same knowledge being requested over and over, use caching. Retail store the outcomes briefly working with tools like Redis or Memcached which means you don’t should repeat expensive operations.
Also, batch your databases functions after you can. Rather than updating a row one by one, update them in groups. This cuts down on overhead and would make your application more efficient.
Remember to examination with massive datasets. Code and queries that work good with one hundred data could possibly crash when they have to handle 1 million.
In brief, scalable apps are quickly apps. Maintain your code restricted, your queries lean, and use caching when essential. These methods enable your software stay smooth and responsive, at the same time as the load increases.
Leverage Load Balancing and Caching
As your app grows, it's got to take care of more users and more visitors. If every thing goes via 1 server, it'll speedily turn into a bottleneck. That’s in which load balancing and caching are available in. These two tools help keep the application rapidly, steady, and scalable.
Load balancing spreads incoming site visitors across various servers. In lieu of just one server doing many of the get the job done, the load balancer routes end users to distinct servers according to availability. This means no one server will get overloaded. If 1 server goes down, the load balancer can send visitors to the Other individuals. Tools like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to put in place.
Caching is about storing info temporarily so it could be reused swiftly. When users ask for exactly the same information yet again—like a product web site or possibly a profile—you don’t have to fetch it within the database when. You could serve it from the cache.
There are 2 common sorts of caching:
1. Server-aspect caching (like Redis or Memcached) shops facts in memory for quick obtain.
2. Customer-side caching (like browser caching or CDN caching) merchants static data files near the user.
Caching lowers databases load, enhances velocity, and tends to make your application more productive.
Use caching for things which don’t modify generally. And usually ensure that your cache is updated when info does improve.
Briefly, load balancing and caching are simple but strong applications. With each other, they help your application handle a lot more people, stay quick, and Get well from complications. If you plan to increase, you would like each.
Use Cloud and Container Applications
To build scalable programs, you may need applications that permit your app develop very easily. That’s wherever cloud website platforms and containers are available. They offer you flexibility, decrease setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World wide web Products and services (AWS), Google Cloud System (GCP), and Microsoft Azure Allow you to rent servers and companies as you may need them. You don’t should invest in components or guess upcoming potential. When traffic increases, you can add much more sources with just a few clicks or immediately utilizing auto-scaling. When targeted traffic drops, it is possible to scale down to save cash.
These platforms also provide solutions like managed databases, storage, load balancing, and security applications. You could deal with making your application as opposed to handling infrastructure.
Containers are An additional key Software. A container offers your app and every thing it needs to run—code, libraries, configurations—into just one unit. This makes it easy to maneuver your app between environments, from a laptop computer towards the cloud, without surprises. Docker is the preferred Device for this.
When your application employs several containers, tools like Kubernetes make it easier to regulate them. Kubernetes handles deployment, scaling, and recovery. If one aspect of the application crashes, it restarts it routinely.
Containers also allow it to be straightforward to individual parts of your application into solutions. You could update or scale elements independently, which is great for performance and dependability.
In short, working with cloud and container tools means it is possible to scale fast, deploy simply, and recover speedily when problems come about. If you want your app to improve with out boundaries, start making use of these applications early. They conserve time, lessen hazard, and enable you to keep centered on developing, not repairing.
Observe Every little thing
When you don’t monitor your application, you gained’t know when points go Completely wrong. Monitoring aids the thing is how your application is performing, spot concerns early, and make greater conclusions as your application grows. It’s a key Portion of constructing scalable devices.
Start by monitoring primary metrics like CPU use, memory, disk House, and response time. These tell you how your servers and solutions are carrying out. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you obtain and visualize this details.
Don’t just monitor your servers—monitor your app too. Keep an eye on how long it will take for consumers to load webpages, how often mistakes take place, and in which they take place. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s occurring within your code.
Put in place alerts for critical troubles. By way of example, When your response time goes over a limit or simply a company goes down, it is best to get notified promptly. This will help you resolve concerns quick, often right before people even observe.
Monitoring is also practical when you make variations. When you deploy a whole new function and find out a spike in glitches or slowdowns, you'll be able to roll it back in advance of it triggers genuine destruction.
As your app grows, traffic and details enhance. With out checking, you’ll skip indications of problems until it’s far too late. But with the correct tools in position, you stay on top of things.
In brief, checking aids you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about comprehending your procedure and ensuring it really works nicely, even stressed.
Final Ideas
Scalability isn’t only for large corporations. Even little applications need a powerful Basis. By creating meticulously, optimizing wisely, and using the suitable tools, it is possible to build applications that mature easily with no breaking stressed. Begin modest, think large, and Make smart.